Matplotlib 图表
显示 Matplotlib 图表。
示例
条形颜色图表
以下示例基于 Matplotlib 文档中的原始示例。
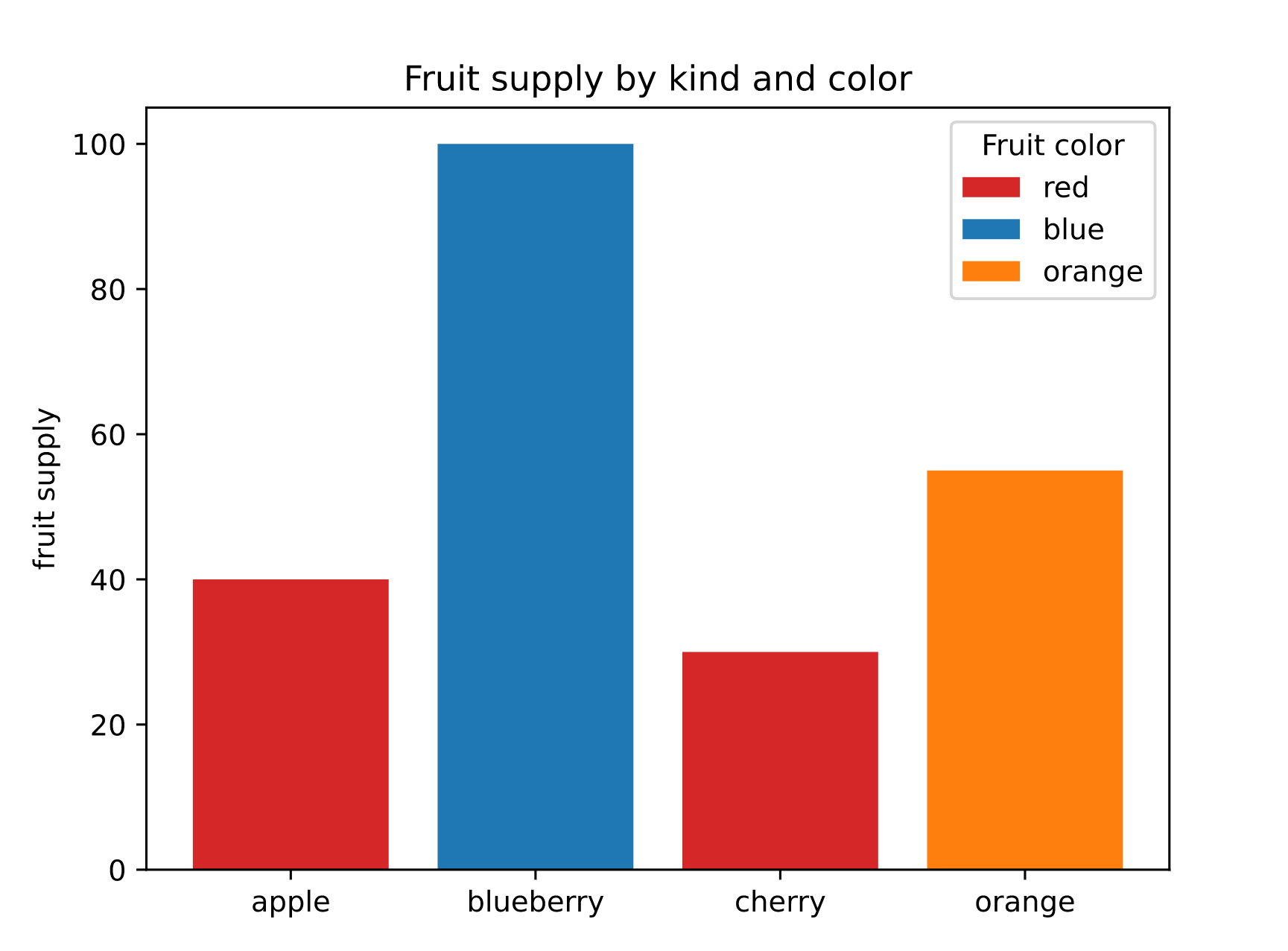
import matplotlib
import matplotlib.pyplot as plt
import flet as ft
from flet.matplotlib_chart import MatplotlibChart
matplotlib.use("svg")
def main(page: ft.Page):
fig, ax = plt.subplots()
fruits = ["apple", "blueberry", "cherry", "orange"]
counts = [40, 100, 30, 55]
bar_labels = ["red", "blue", "_red", "orange"]
bar_colors = ["tab:red", "tab:blue", "tab:red", "tab:orange"]
ax.bar(fruits, counts, label=bar_labels, color=bar_colors)
ax.set_ylabel("fruit supply")
ax.set_title("Fruit supply by kind and color")
ax.legend(title="Fruit color")
page.add(MatplotlibChart(fig, expand=True))
ft.app(target=main)
线图
以下示例基于 Matplotlib 文档中的原始示例。
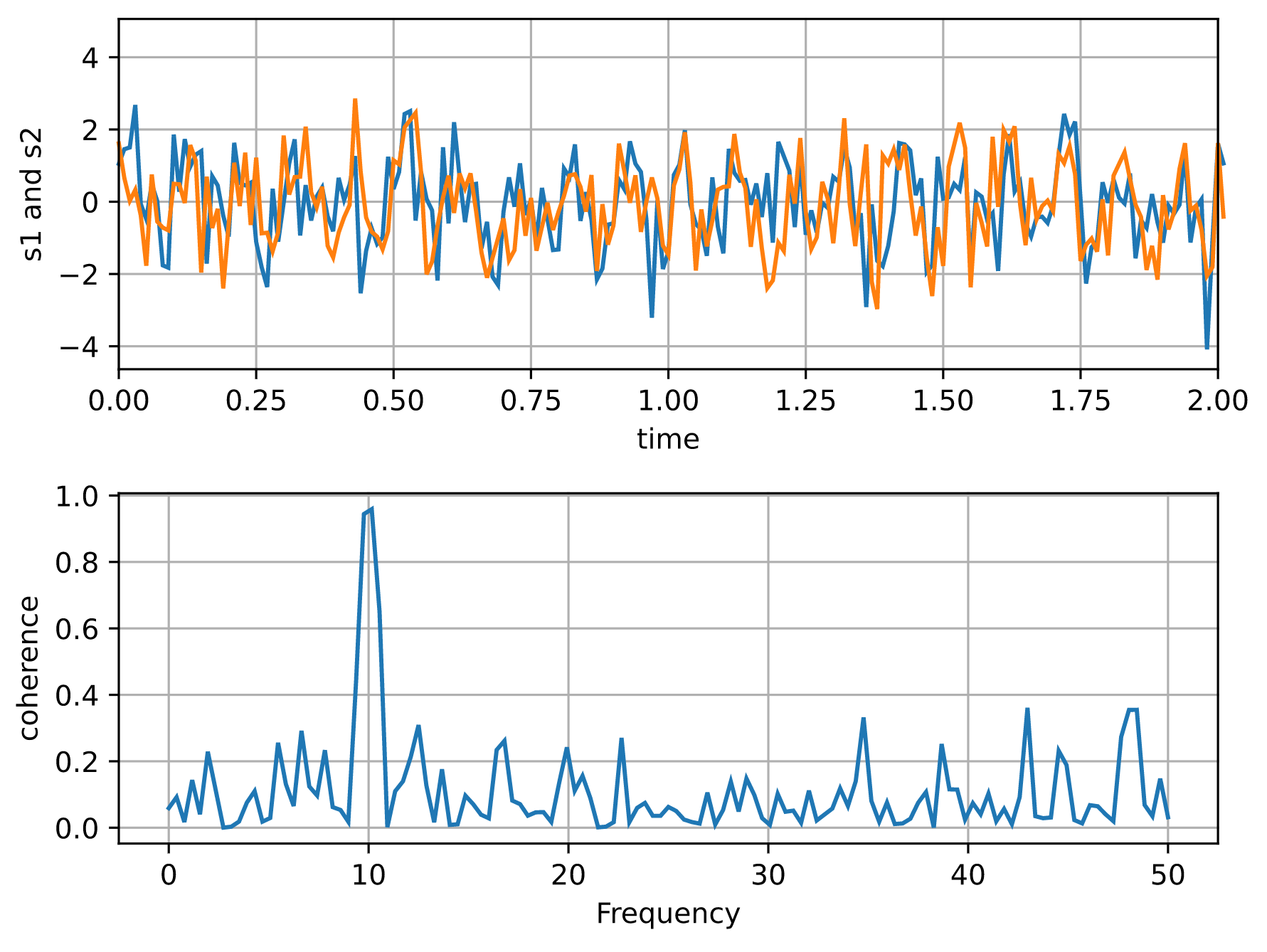
import matplotlib
import matplotlib.pyplot as plt
import numpy as np
import flet as ft
from flet.matplotlib_chart import MatplotlibChart
matplotlib.use("svg")
def main(page: ft.Page):
# Fixing random state for reproducibility
np.random.seed(19680801)
dt = 0.01
t = np.arange(0, 30, dt)
nse1 = np.random.randn(len(t)) # white noise 1
nse2 = np.random.randn(len(t)) # white noise 2
# Two signals with a coherent part at 10Hz and a random part
s1 = np.sin(2 * np.pi * 10 * t) + nse1
s2 = np.sin(2 * np.pi * 10 * t) + nse2
fig, axs = plt.subplots(2, 1)
axs[0].plot(t, s1, t, s2)
axs[0].set_xlim(0, 2)
axs[0].set_xlabel("time")
axs[0].set_ylabel("s1 and s2")
axs[0].grid(True)
cxy, f = axs[1].cohere(s1, s2, 256, 1.0 / dt)
axs[1].set_ylabel("coherence")
fig.tight_layout()
page.add(MatplotlibChart(fig, expand=True))
ft.app(target=main)
属性
figure
要绘制的 Matplotlib 图形 - matplotlib.figure.Figure
类的实例。
isolated
每次更新页面或父控件时,chart 都会被重新绘制,调用 Matplotlib API。这可能会影响 Flet 应用的性能。
将 isolated
设置为 True
,以启用 explicit chart 更新。要重新绘制 chart,请调用其 update()
方法。例如,第一个示例可以修改如下:
def main(page: ft.Page):
#...
# 设置初始轴 legend
ax.legend(title="Fruit color")
# 启用 explicit 更新
# 并将 chart 添加到页面
chart1 = MatplotlibChart(fig, isolated=True, expand=True)
page.add(chart1)
sleep(5)
# 更新 chart 轴
ax.legend(title="Colors")
chart1.update()
ft.app(target=main)
original_size
True
以原始大小显示 chart。False
(默认) 以配置的边界显示 chart。
transparent
True
从 chart 中删除背景。False
(默认) 以背景显示 chart。