折线图 LineChart
绘制折线图。
示例
折线图 1
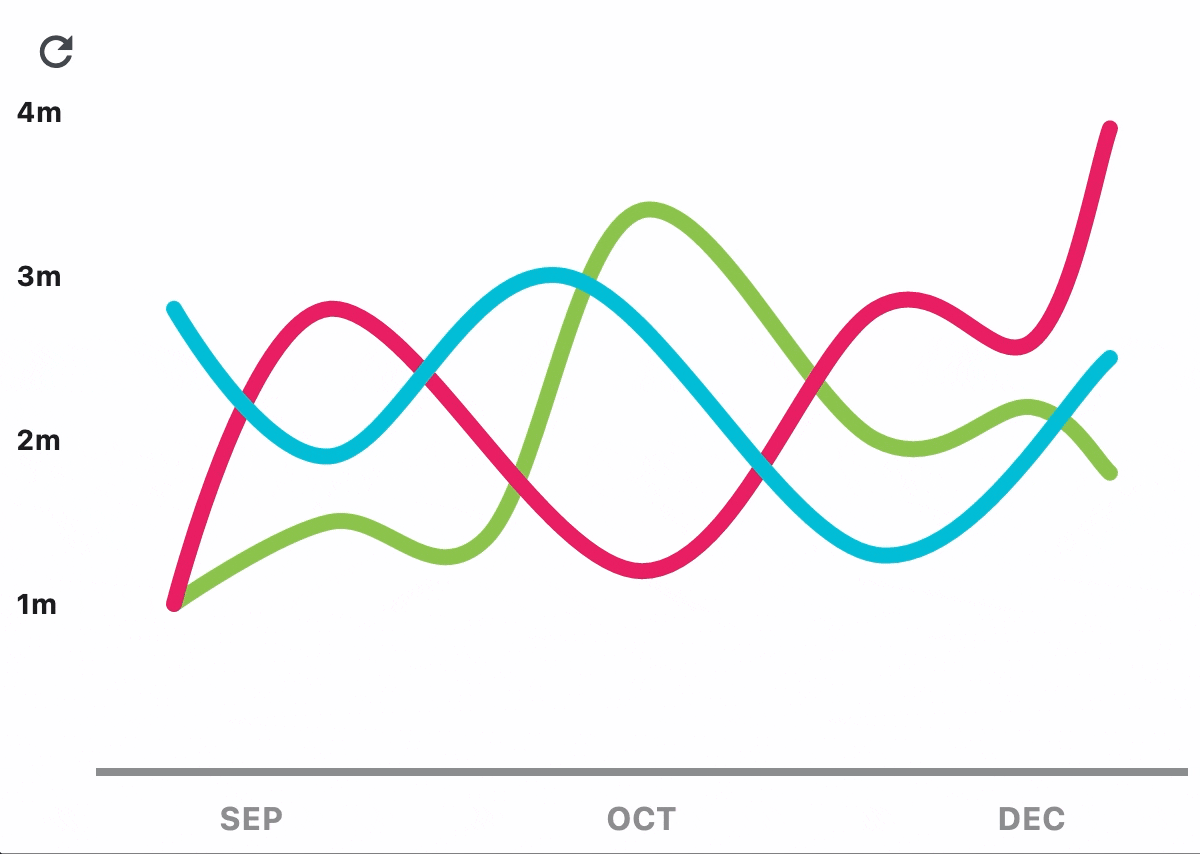
import flet as ft
class State:
toggle = True
s = State()
def main(page: ft.Page):
data_1 = [
ft.LineChartData(
data_points=[
ft.LineChartDataPoint(1, 1),
ft.LineChartDataPoint(3, 1.5),
ft.LineChartDataPoint(5, 1.4),
ft.LineChartDataPoint(7, 3.4),
ft.LineChartDataPoint(10, 2),
ft.LineChartDataPoint(12, 2.2),
ft.LineChartDataPoint(13, 1.8),
],
stroke_width=8,
color=ft.colors.LIGHT_GREEN,
curved=True,
stroke_cap_round=True,
),
ft.LineChartData(
data_points=[
ft.LineChartDataPoint(1, 1),
ft.LineChartDataPoint(3, 2.8),
ft.LineChartDataPoint(7, 1.2),
ft.LineChartDataPoint(10, 2.8),
ft.LineChartDataPoint(12, 2.6),
ft.LineChartDataPoint(13, 3.9),
],
color=ft.colors.PINK,
below_line_bgcolor=ft.colors.with_opacity(0, ft.colors.PINK),
stroke_width=8,
curved=True,
stroke_cap_round=True,
),
ft.LineChartData(
data_points=[
ft.LineChartDataPoint(1, 2.8),
ft.LineChartDataPoint(3, 1.9),
ft.LineChartDataPoint(6, 3),
ft.LineChartDataPoint(10, 1.3),
ft.LineChartDataPoint(13, 2.5),
],
color=ft.colors.CYAN,
stroke_width=8,
curved=True,
stroke_cap_round=True,
),
]
data_2 = [
ft.LineChartData(
data_points=[
ft.LineChartDataPoint(1, 1),
ft.LineChartDataPoint(3, 4),
ft.LineChartDataPoint(5, 1.8),
ft.LineChartDataPoint(7, 5),
ft.LineChartDataPoint(10, 2),
ft.LineChartDataPoint(12, 2.2),
ft.LineChartDataPoint(13, 1.8),
],
stroke_width=4,
color=ft.colors.with_opacity(0.5, ft.colors.LIGHT_GREEN),
stroke_cap_round=True,
),
ft.LineChartData(
data_points=[
ft.LineChartDataPoint(1, 1),
ft.LineChartDataPoint(3, 2.8),
ft.LineChartDataPoint(7, 1.2),
ft.LineChartDataPoint(10, 2.8),
ft.LineChartDataPoint(12, 2.6),
ft.LineChartDataPoint(13, 3.9),
],
color=ft.colors.with_opacity(0.5, ft.colors.PINK),
below_line_bgcolor=ft.colors.with_opacity(0.2, ft.colors.PINK),
stroke_width=4,
curved=True,
stroke_cap_round=True,
),
ft.LineChartData(
data_points=[
ft.LineChartDataPoint(1, 3.8),
ft.LineChartDataPoint(3, 1.9),
ft.LineChartDataPoint(6, 5),
ft.LineChartDataPoint(10, 3.3),
ft.LineChartDataPoint(13, 4.5),
],
color=ft.colors.with_opacity(0.5, ft.colors.CYAN),
stroke_width=4,
stroke_cap_round=True,
),
]
chart = ft.LineChart(
data_series=data_1,
border=ft.Border(
bottom=ft.BorderSide(4, ft.colors.with_opacity(0.5, ft.colors.ON_SURFACE))
),
left_axis=ft.ChartAxis(
labels=[
ft.ChartAxisLabel(
value=1,
label=ft.Text("1m", size=14, weight=ft.FontWeight.BOLD),
),
ft.ChartAxisLabel(
value=2,
label=ft.Text("2m", size=14, weight=ft.FontWeight.BOLD),
),
ft.ChartAxisLabel(
value=3,
label=ft.Text("3m", size=14, weight=ft.FontWeight.BOLD),
),
ft.ChartAxisLabel(
value=4,
label=ft.Text("4m", size=14, weight=ft.FontWeight.BOLD),
),
ft.ChartAxisLabel(
value=5,
label=ft.Text("5m", size=14, weight=ft.FontWeight.BOLD),
),
ft.ChartAxisLabel(
value=6,
label=ft.Text("6m", size=14, weight=ft.FontWeight.BOLD),
),
],
labels_size=40,
),
bottom_axis=ft.ChartAxis(
labels=[
ft.ChartAxisLabel(
value=2,
label=ft.Container(
ft.Text(
"SEP",
size=16,
weight=ft.FontWeight.BOLD,
color=ft.colors.with_opacity(0.5, ft.colors.ON_SURFACE),
),
margin=ft.margin.only(top=10),
),
),
ft.ChartAxisLabel(
value=7,
label=ft.Container(
ft.Text(
"OCT",
size=16,
weight=ft.FontWeight.BOLD,
color=ft.colors.with_opacity(0.5, ft.colors.ON_SURFACE),
),
margin=ft.margin.only(top=10),
),
),
ft.ChartAxisLabel(
value=12,
label=ft.Container(
ft.Text(
"DEC",
size=16,
weight=ft.FontWeight.BOLD,
color=ft.colors.with_opacity(0.5, ft.colors.ON_SURFACE),
),
margin=ft.margin.only(top=10),
),
),
],
labels_size=32,
),
tooltip_bgcolor=ft.colors.with_opacity(0.8, ft.colors.BLUE_GREY),
min_y=0,
max_y=4,
min_x=0,
max_x=14,
# animate=5000,
expand=True,
)
def toggle_data(e):
if s.toggle:
chart.data_series = data_2
chart.data_series[2].point = True
chart.max_y = 6
chart.interactive = False
else:
chart.data_series = data_1
chart.max_y = 4
chart.interactive = True
s.toggle = not s.toggle
chart.update()
page.add(ft.IconButton(ft.icons.REFRESH, on_click=toggle_data), chart)
ft.app(main)
LineChart 2
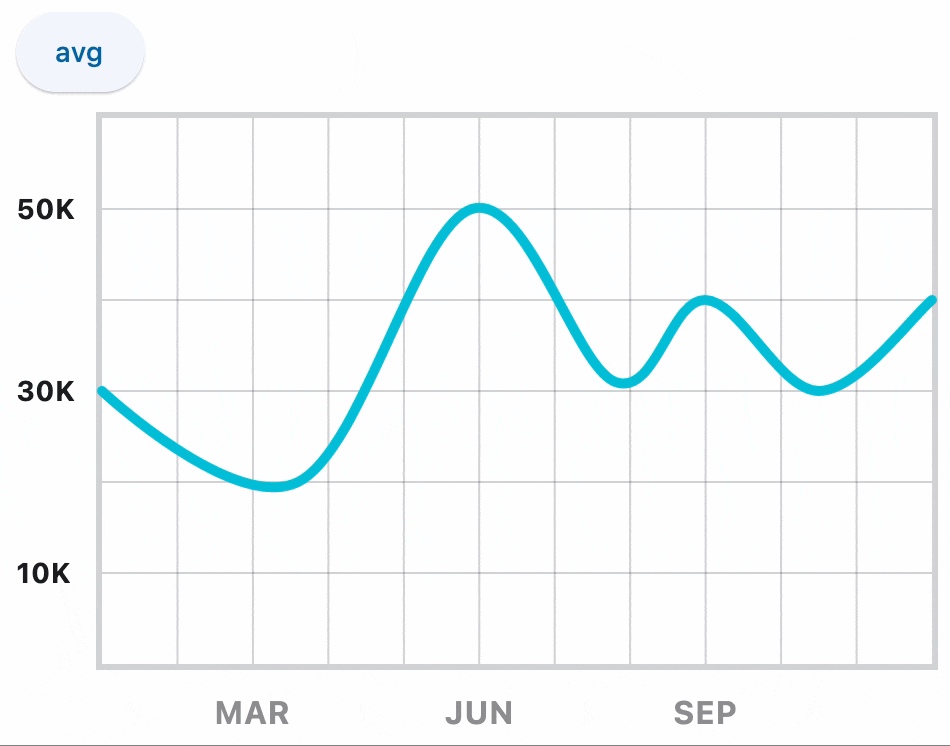
import flet as ft
class State:
toggle = True
s = State()
def main(page: ft.Page):
data_1 = [
ft.LineChartData(
data_points=[
ft.LineChartDataPoint(0, 3),
ft.LineChartDataPoint(2.6, 2),
ft.LineChartDataPoint(4.9, 5),
ft.LineChartDataPoint(6.8, 3.1),
ft.LineChartDataPoint(8, 4),
ft.LineChartDataPoint(9.5, 3),
ft.LineChartDataPoint(11, 4),
],
stroke_width=5,
color=ft.colors.CYAN,
curved=True,
stroke_cap_round=True,
)
]
data_2 = [
ft.LineChartData(
data_points=[
ft.LineChartDataPoint(0, 3.44),
ft.LineChartDataPoint(2.6, 3.44),
ft.LineChartDataPoint(4.9, 3.44),
ft.LineChartDataPoint(6.8, 3.44),
ft.LineChartDataPoint(8, 3.44),
ft.LineChartDataPoint(9.5, 3.44),
ft.LineChartDataPoint(11, 3.44),
],
stroke_width=5,
color=ft.colors.CYAN,
curved=True,
stroke_cap_round=True,
)
]
chart = ft.LineChart(
data_series=data_1,
border=ft.border.all(3, ft.colors.with_opacity(0.2, ft.colors.ON_SURFACE)),
horizontal_grid_lines=ft.ChartGridLines(
interval=1, color=ft.colors.with_opacity(0.2, ft.colors.ON_SURFACE), width=1
),
vertical_grid_lines=ft.ChartGridLines(
interval=1, color=ft.colors.with_opacity(0.2, ft.colors.ON_SURFACE), width=1
),
left_axis=ft.ChartAxis(
labels=[
ft.ChartAxisLabel(
value=1,
label=ft.Text("10K", size=14, weight=ft.FontWeight.BOLD),
),
ft.ChartAxisLabel(
value=3,
label=ft.Text("30K", size=14, weight=ft.FontWeight.BOLD),
),
ft.ChartAxisLabel(
value=5,
label=ft.Text("50K", size=14, weight=ft.FontWeight.BOLD),
),
],
labels_size=40,
),
bottom_axis=ft.ChartAxis(
labels=[
ft.ChartAxisLabel(
value=2,
label=ft.Container(
ft.Text(
"MAR",
size=16,
weight=ft.FontWeight.BOLD,
color=ft.colors.with_opacity(0.5, ft.colors.ON_SURFACE),
),
margin=ft.margin.only(top=10),
),
),
ft.ChartAxisLabel(
value=5,
label=ft.Container(
ft.Text(
"JUN",
size=16,
weight=ft.FontWeight.BOLD,
color=ft.colors.with_opacity(0.5, ft.colors.ON_SURFACE),
),
margin=ft.margin.only(top=10),
),
),
ft.ChartAxisLabel(
value=8,
label=ft.Container(
ft.Text(
"SEP",
size=16,
weight=ft.FontWeight.BOLD,
color=ft.colors.with_opacity(0.5, ft.colors.ON_SURFACE),
),
margin=ft.margin.only(top=10),
),
),
],
labels_size=32,
),
tooltip_bgcolor=ft.colors.with_opacity(0.8, ft.colors.BLUE_GREY),
min_y=0,
max_y=6,
min_x=0,
max_x=11,
# animate=5000,
expand=True,
)
def toggle_data(e):
if s.toggle:
chart.data_series = data_2
chart.interactive = False
else:
chart.data_series = data_1
chart.interactive = True
s.toggle = not s.toggle
chart.update()
page.add(ft.ElevatedButton("avg", on_click=toggle_data), chart)
ft.app(main)
LineChart
属性
data_series
作为单独线条在图表上绘制的 LineChartData
控件的列表。
animate
控制图表的隐式动画。
animate
属性的值可以是以下类型之一:
bool
-True
启用使用线性曲线和持续时间为1000
毫秒的图表动画。int
- 启用使用线性曲线和指定毫秒数的图表动画。ft.Animation(duration: int, curve: str)
- 启用使用指定持续时间和过渡曲线的图表动画。
如果 animate
为 None
,则默认情况下启用持续时间为 150
毫秒的线性动画。
baseline_x
Baseline value for X axis. Default is 0
.
baseline_y
Baseline value for Y axis. Default is 0
.
interactive
在悬停在图表上时启用自动工具提示和点高亮显示。
bgcolor
图表的背景颜色。
tooltip_bgcolor
工具提示的背景颜色。
border
图表周围的边框。该值是 ft.Border
类的实例。
point_line_start
绘制在选定点下方的垂直线的起始位置。默认为图表的底部边缘。
point_line_end
绘制在选定点位置的垂直线的结束位置。默认为数据点的 y
值。
horizontal_grid_lines
控制图表的水平线的绘制。该值是 ChartGridLines
类的实例。
vertical_grid_lines
控制图表的垂直线的绘制。该值是 ChartGridLines
类的实例。
left_axis
配置左轴的外观、标题和标签。该值是 ChartAxis
类的实例。
tooltip_border_side
工具提示边框边。
tooltip_direction
控制在顶部或底部显示工具提示。值的类型为TooltipDirection
,默认为TooltipDirection.AUTO
。
tooltip_fit_inside_horizontally
如果发生溢出,强制工具提示在图表内水平移动。值的类型为bool
。
tooltip_fit_inside_vertically
如果发生溢出,强制工具提示在图表内垂直移动。值的类型为bool
。
tooltip_horizontal_offset
应用水平偏移以显示工具提示。
tooltip_margin
在棒图顶部显示工具提示时应用底部边距。
tooltip_max_content_width
限制工具提示的宽度。
tooltip_padding
应用填充以在工具提示内显示内容。
tooltip_rounded_radius
为工具提示设置圆角半径。
tooltip_rotate_angle
工具提示的旋转角度。
tooltip_show_on_top_of_chart_box_area
强制工具提示容器位于线条顶部。值的类型为bool
,默认为False
。
top_axis
配置顶部轴的外观、标题和标签。该值是 ChartAxis
类的实例。
right_axis
配置右轴的外观、标题和标签。该值是 ChartAxis
类的实例。
bottom_axis
配置底部轴的外观、标题和标签。该值是 ChartAxis
类的实例。
baseline_x
X 轴的基准值。默认为 0
。
min_x
配置 X 轴的最小显示值。
max_x
配置 X 轴的最大显示值。
baseline_y
Y 轴的基准值。默认为 0
。
min_y
配置 Y 轴的最小显示值。
max_y
配置 Y 轴的最大显示值。
LineChart
事件
on_chart_event
当悬停或点击图表线条时触发。
事件数据是 ft.LineChartEvent
类的实例,具有以下属性:
type
- 事件类型,例如PointerHoverEvent
、PointerExitEvent
等。bar_index
- 线条的索引,如果没有悬停在线条上,则为-1
。spot_index
- 线条点的索引,如果没有悬停在点上,则为-1
。
LineChartData
属性
data_points
表示单个图表线条的 LineChartDataPoint
类型的点(圆点)列表。
curved
设置为 True
以绘制曲线状的图表线条。默认为 False
。
color
图表线条的颜色。
gradient
绘制线条背景的渐变。有关更多信息和可能的值,请参阅 Gradient
属性。
stroke_width
图表线条的宽度。
stroke_cap_round
设置为 True
以绘制圆角线帽。默认为 False
。
dash_pattern
定义线条的虚线效果。该值是一个循环列表,其中包含虚线偏移和长度。例如,列表 [5, 10]
将绘制长度为 5 像素的虚线,后跟长度为 10 像素的空白区域。默认情况下,绘制实线。
shadow
线条投射的阴影。该值是 ft.Shadow
类的实例。
above_line_bgcolor
使用指定的颜色填充图表线条上方的区域。
above_line_gradient
使用指定的渐变填充图表线条上方的区域。
above_line_cutoff_y
在特定 Y 值处截断填充的线条上方区域。
above_line
在线条点和图表顶部边缘之间绘制的垂直线。该值是 ChartPointLine
类的实例。
below_line_bgcolor
使用指定的颜色填充图表线条下方的区域。
below_line_gradient
使用指定的渐变填充图表线条下方的区域。
below_line_cutoff_y
在特定 Y 值处截断填充的线条下方区域。
below_line
在 线条点和图表底部边缘之间绘制的垂直线。该值是 ChartPointLine
类的实例。
selected_below_line
在选定的线条点和图表底部边缘之间绘制的垂直线。该值可以是 True
- 使用默认样式绘制线条,False
- 不在选定点下方绘制线条,或者是 ChartPointLine
类的实例,用于指定要绘制的线条样式。
point
配置线条点(圆点)的外观和形状。该属性的值可以是 True
- 使用默认样式绘制点,False
- 不绘制线条点,或者是 ChartPointShape
类的实现之一:
ChartCirclePoint
- 圆形点ChartSquarePoint
- 方形点ChartCrossPoint
- 十字点
selected_point
配置选定线条点的外观和形状。有关支持的属性值,请参阅 LineChartData.point
。