导航抽屉
Material Design 导航抽屉组件。
导航抽屉是一个水平从页面左或右边缘滑入的面板,用于显示应用程序的主要目的地。要将导航抽屉添加到页面,请使用 page.drawer
和 page.end_drawer
属性。类似地,导航抽屉也可以添加到 View
中。要显示抽屉,请将其 open
属性设置为 True
。
要打开此控件,只需调用page.open()
辅助方法。
示例
从页面左边缘滑入的导航抽屉
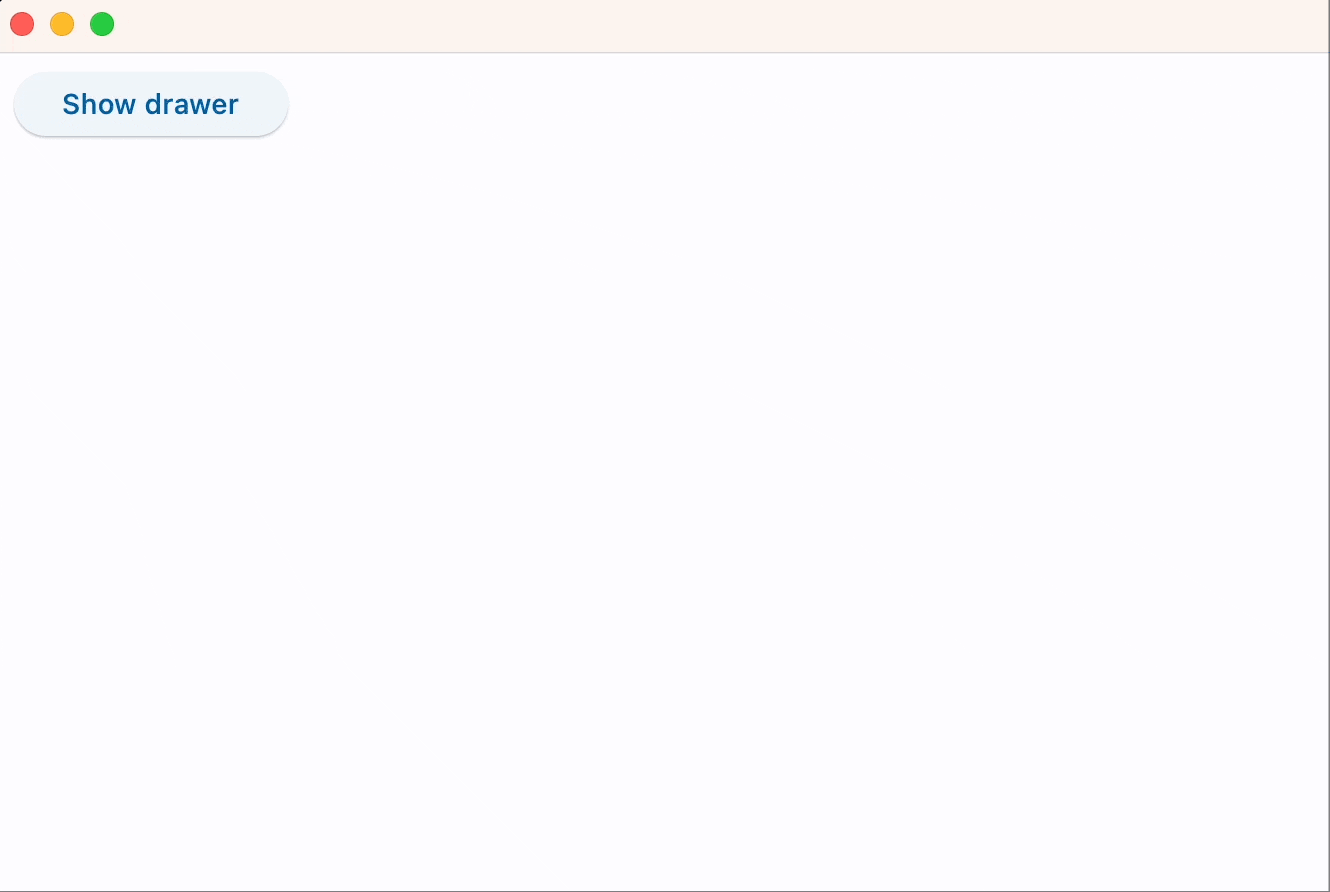
import flet as ft
def main(page: ft.Page):
page.horizontal_alignment = ft.CrossAxisAlignment.CENTER
def handle_dismissal(e):
page.add(ft.Text("Drawer dismissed"))
def handle_change(e):
page.add(ft.Text(f"Selected Index changed: {e.selected_index}"))
# page.close(drawer)
drawer = ft.NavigationDrawer(
on_dismiss=handle_dismissal,
on_change=handle_change,
controls=[
ft.Container(height=12),
ft.NavigationDrawerDestination(
label="Item 1",
icon=ft.icons.DOOR_BACK_DOOR_OUTLINED,
selected_icon_content=ft.Icon(ft.icons.DOOR_BACK_DOOR),
),
ft.Divider(thickness=2),
ft.NavigationDrawerDestination(
icon_content=ft.Icon(ft.icons.MAIL_OUTLINED),
label="Item 2",
selected_icon=ft.icons.MAIL,
),
ft.NavigationDrawerDestination(
icon_content=ft.Icon(ft.icons.PHONE_OUTLINED),
label="Item 3",
selected_icon=ft.icons.PHONE,
),
],
)
page.add(ft.ElevatedButton("Show drawer", on_click=lambda e: page.open(drawer)))
ft.app(main)
从页面右边缘滑入的导航抽屉
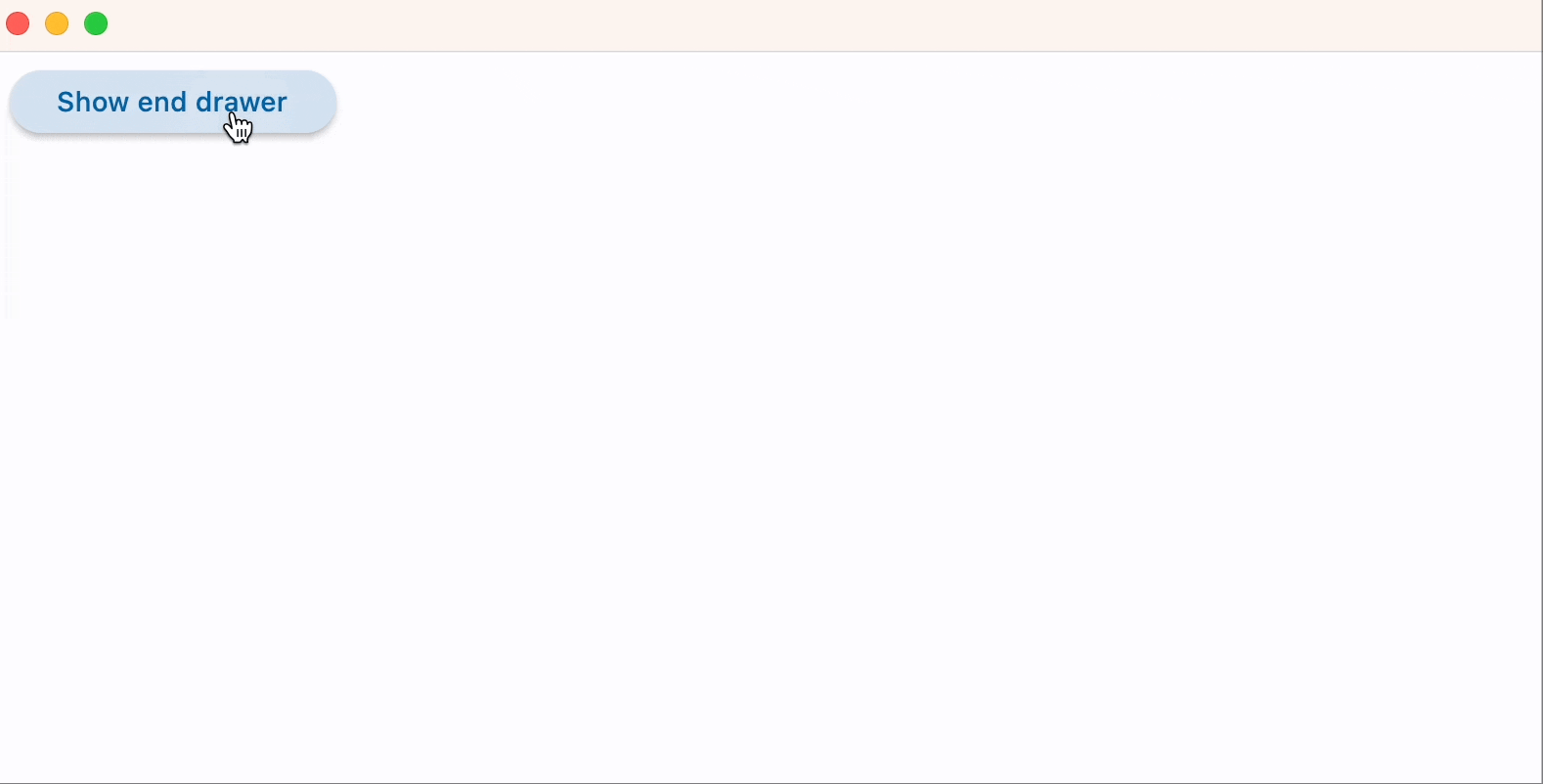
import flet as ft
def main(page: ft.Page):
page.horizontal_alignment = ft.CrossAxisAlignment.CENTER
def handle_dismissal(e):
page.add(ft.Text("End drawer dismissed"))
def handle_change(e):
page.add(ft.Text(f"Selected Index changed: {e.control.selected_index}"))
# page.close(end_drawer)
end_drawer = ft.NavigationDrawer(
position=ft.NavigationDrawerPosition.END,
on_dismiss=handle_dismissal,
on_change=handle_change,
controls=[
ft.NavigationDrawerDestination(icon=ft.icons.ADD_TO_HOME_SCREEN_SHARP, label="Item 1"),
ft.NavigationDrawerDestination(icon=ft.icons.ADD_COMMENT, label="Item 2"),
],
)
page.add(ft.ElevatedButton("Show end drawer", on_click=lambda e: page.open(end_drawer)))
ft.app(main)
NavigationDrawer
属性
bgcolor
导航抽屉的背景颜色。
controls
定义导航抽屉中的项目外观。
列表包含 NavigationDrawerDestination
项和/或其他控件,如标题和分隔符。
elevation
导航抽屉的高度。
indicator_color
选定目的地指示器的颜色。
indicator_shape
所选目标指示器的形状。
值的类型为 OutlinedBorder
。
position
此抽屉的位置。
值的类型为 NavigationDrawerPosition
,默认值为 NavigationDrawerPosition.START
。
selected_index
当前选定的 NavigationDrawerDestination
的索引,或者如果没有目的地被选定,则为 null。
有效的 selected_index
是一个介于 0 和目的地数量 - 1 之间的整数。对于无效的 selected_index
,例如 -1
,所有目的地将显示未选定。
shadow_color
用于表示高度的阴影颜色。
surface_tint_color
Material 的 surface tint,用于保存导航抽屉的内容。
tile_padding
定义 NavigationDrawerDestination
控件的填充。
NavigationDrawer
事件
on_change
当选定目的地更改时触发。
on_dismiss
当导航抽屉被dismiss(例如,点击外部或programmatically)时触发。
NavigationDrawerDestination
属性
bgcolor
该目的地的背景颜色。
icon
该目的地的图标名称。
icon_content
该目的地的图标控件。通常是 Icon
控件。用于代替 Icon
属性。
如果提供了 selected_icon_content
,则该图标将仅在目的地未被选定时显示。
为使导航抽屉更加可访问,请考虑选择具有描绘和填充版本的图标,例如 icons.CLOUD
和 icons.CLOUD_QUEUE
。图标应设置为描绘版本,而 selected_icon
设置为填充版本。
label
该目的地的文本标签,显示在图标下方。
selected_icon
该目的地被选定时显示的替代图标名称。
selected_icon_content
该目的地被选定时显示的替代图标控件。
如果未提供该图标,则导航抽屉将在任何状态下显示 icon_content
。